100% Open Source: |
Apache License, v2.0 |
Monitoring:
- Web Interface.
- See all running jobs.
- View current status.
|
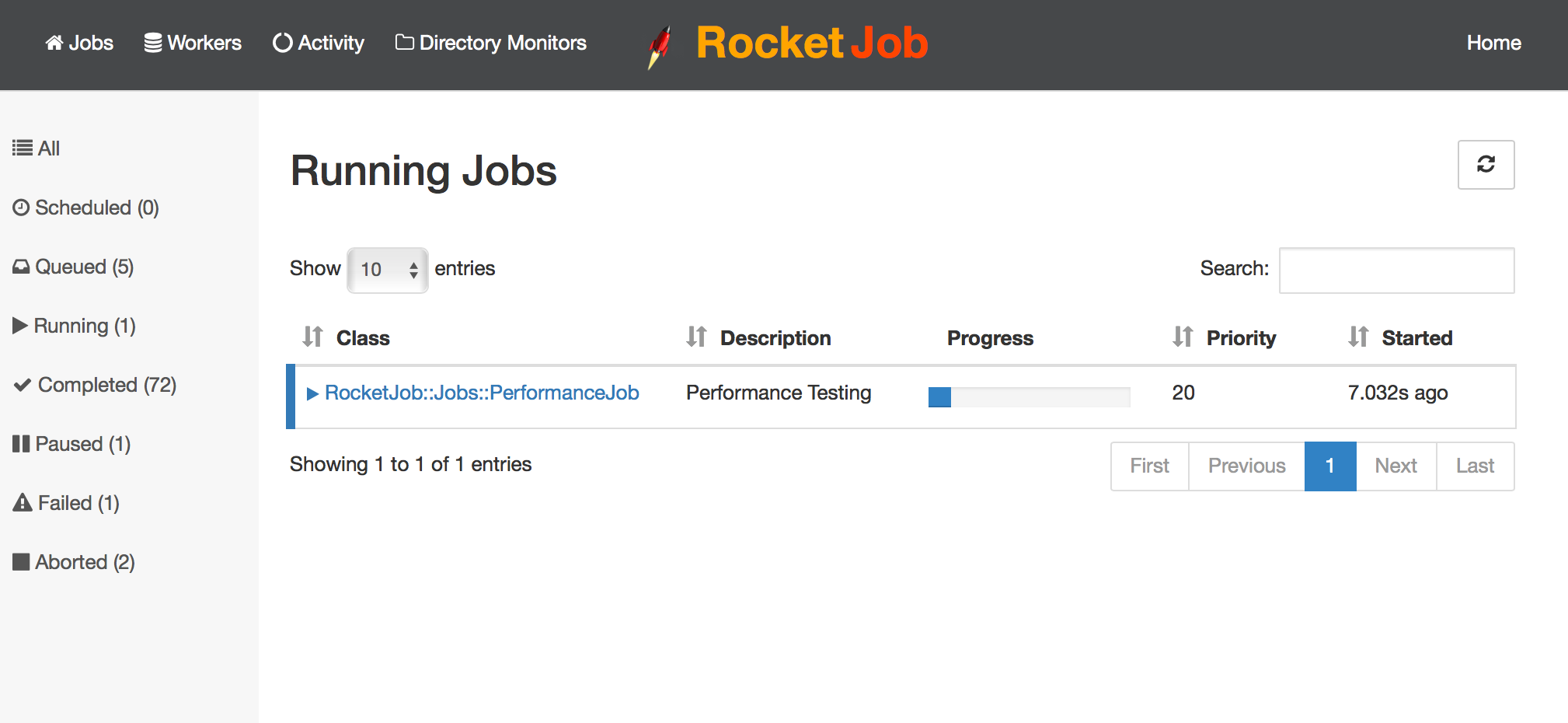 |
Priority based processing.
- Process jobs in business priority order.
- Dynamically change job priority to push through business critical jobs.
|
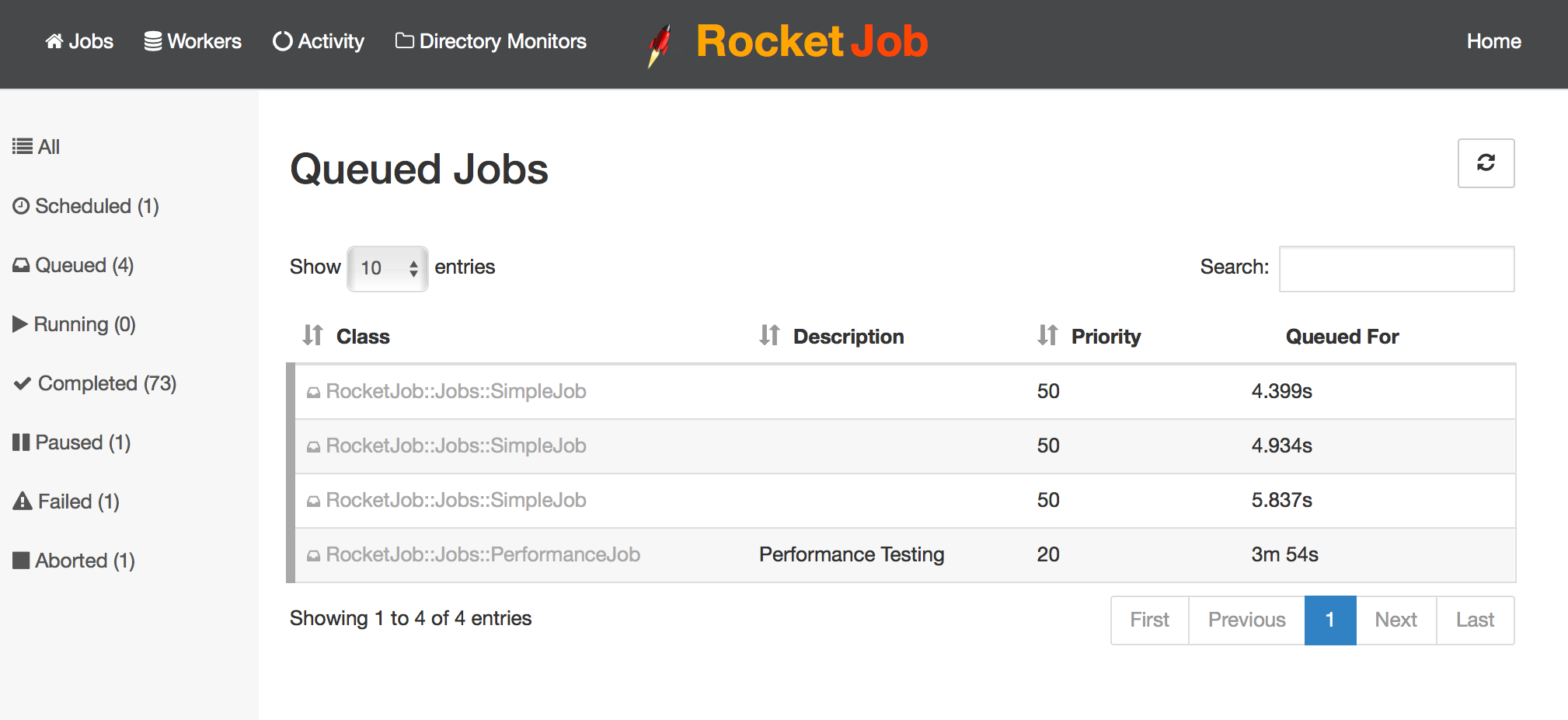 |
Cron replacement.
|
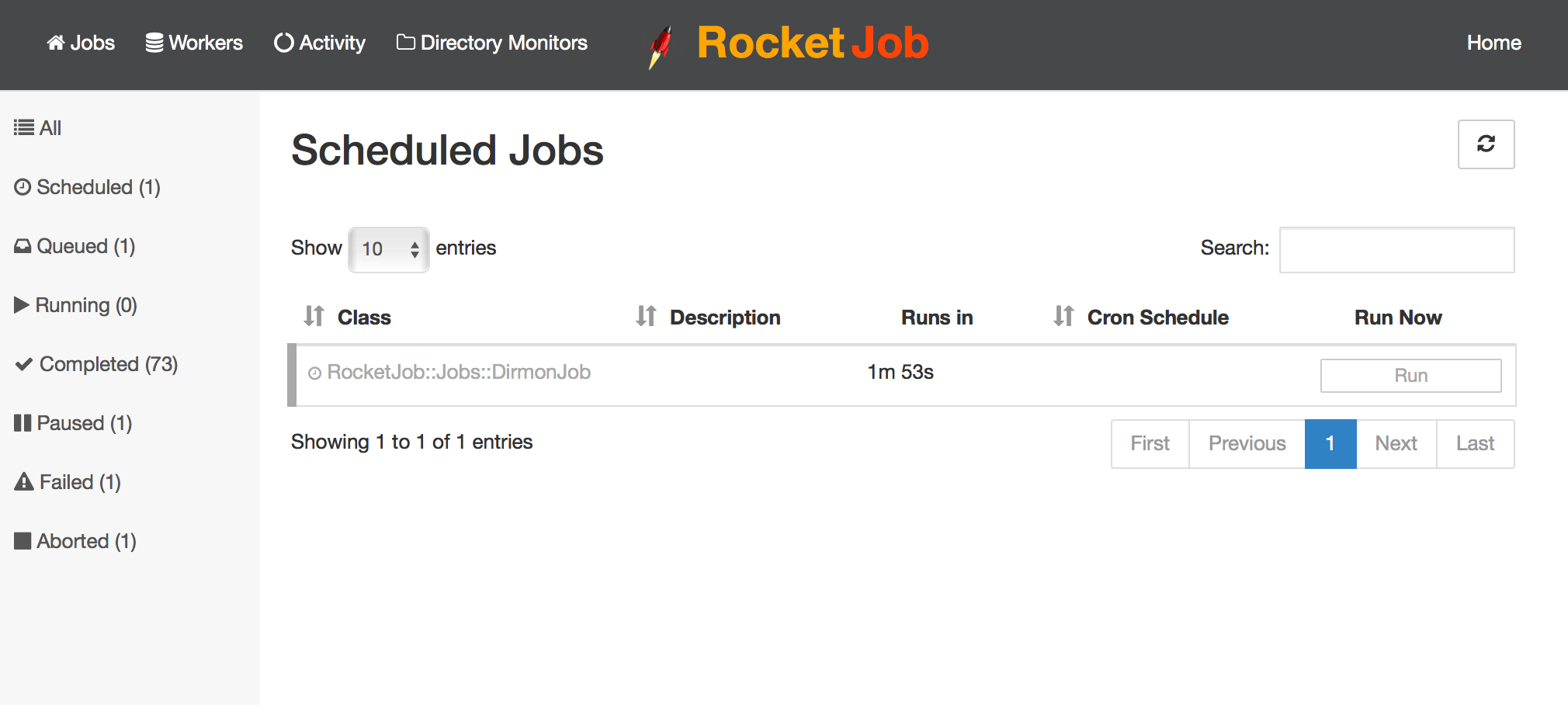 |
Familiar interface.
|
ImportJob.create!(
file_name: "file.csv",
priority: 5
)
|
Implement behavior.
|
class MyJob < RocketJob::Job
def perform
# Implement behavior here ...
end
end
|
Fields, with types:
- Array
- Date
- Float
- Hash
- Integer
- Range
- Regexp
- String
- Time
- Etc.
|
class Job < RocketJob::Job
field :login, String
field :count, Integer
def perform
# Implement behavior here ...
end
end
|
Validations:
|
class Job < RocketJob::Job
field :login, String
field :count, Integer
def perform
# Implement behavior here ...
end
validates_presence_of :login
validates :count, inclusion: 1..100
end
|
Callbacks:
- Extensive callback hooks to customize job processing and behavior.
|
class MyJob < RocketJob::Job
after_start :email_started
def perform
# Implement behavior here ...
end
# Send an email when the job starts
def email_started
MyJobMailer.started(self).deliver_now
end
end
|
Batch Jobs:
- Use many workers to process a single job at the same time.
- Process large files by breaking it up into pieces that can be processed concurrently across all available workers.
|
class ImportPricesJob < RocketJob::Job
include RocketJob::Batch

def perform(row)
# Each row is a Hash
# Load into DB via Active Record
Price.create!(row)
end
end
# Upload the file into the Batch Job for processing
job = ImportPricesJob.new
job.upload("prices.csv")
job.save!
|
Trigger Jobs when new files arrive:
- Dirmon monitors directories for new files.
- Kicks off a Rocket Job to process the file.
|
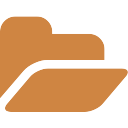 |
High Performance:
- Over 1,000 jobs per second on a single server.
|
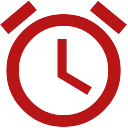 |
Reliable:
- Reliably process and track every job.
|
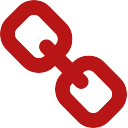 |
Scalable:
- Scales from a single worker to thousands of workers across hundreds of servers.
|
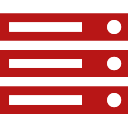 |
High Performance Metrics and Logging:
- File
- Bugsnag
- Apache Kafka
- ElasticSearch
- MongoDB
- NewRelic
- Splunk
- Syslog (TCP, UDP, & local)
- Etc.
|
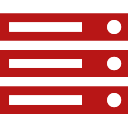 |
Fully supports and integrates with Rails.
|
 |